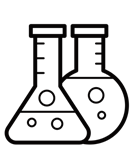
Chemistry APIs
A collection of chemistry REST APIs that provide detailed calculations for chemistry related formulas
GET /++getChemElement
Description
The /++getChemElement
service handles requests to retrieve comprehensive information about a specified chemical element. It processes the input, retrieves element properties, and formats the response.
Request Parameters
- element (string, required): The symbol of the chemical element.
Responses
- 200 OK: Returns a JSON object with the element's properties.
- 400 Bad Request: Missing required parameters.
- 500 Internal Server Error: An unknown error occurred.
Example
{
"Element": {
"name": "Silver",
"symbol": "Ag",
"atomicNumber": 47,
"group": 11,
"period": 5,
"phase": "solid",
"discoveredBy": "Prehistoric",
"year": null
},
"Atomic Properties": {
"atomicMass": 107.868,
"atomicRadius": 1.8,
"density": 10.5,
"electronegativity": 1.93,
"firstIonization": 7.5762,
"isotopes": 27,
"massNumber": 108,
"neutrons": 61,
"protons": 47
},
"Physical Properties": {
"meltingPoint": 1234.15,
"boilingPoint": 2435,
"specificHeat": 0.235
},
"Chemical Properties": {
"config": [
"[Kr] 4d10 5s1"
],
"metal": true,
"metalloid": false,
"nonmetal": false,
"radioactive": false,
"natural": true,
"valence": 0,
"shells": 5,
"type": "Transition Metal"
}
}
GET /++makeChemCompound
Description
The /++makeChemCompound
endpoint handles a request to compute details about a chemical compound, including its formula, occurrences, molar mass, and percentage composition by mass of its constituent elements. It supports input parameters for the compound's weight, moles, or number of molecules but only one of these measurements is allowed at a time.
Depending on the provided measurement parameter (weight
, moles
, molecules
), the API computes the stoichiometric amounts of the Compound
. So, compound stoichiometric amounts
may or may not be in the response
Request Parameters
- compound (string, required): The formula of the chemical compound (e.g. H2O).
- weight (string, optional): The weight of the compound in grams (e.g. 2).
- moles (string, optional): The amount of the compound in moles (e.g. 2).
- molecules (string, optional): The number of molecules of the compound (e.g. 2e+24).
Responses
- 200 OK: Returns a JSON object with compound information.
- 400 Bad Request: Missing required parameters.
- 401 Unauthorized: More than one measurement provided.
- 500 Internal Server Error: An unknown error occurred.
Example
{
"formula": "H₂O₁",
"occurrences": {
"H": 2,
"O": 1
},
"molar mass (g/mol)": 18.015,
"percent of element in compound": {
"H": 11.19067443796836,
"O": 88.80932556203163
},
"stoichiometric amounts": {
"grams": 2,
"molecules": 6.683319456008882e+22,
"moles": 0.11101859561476547
}
}
POST /++getChemEfPercentComposition
Description
This endpoint handles requests for calculating the empirical formula based on input data. It first validates the subscription status of the request and then processes the input data to compute the empirical formula. The endpoint also includes error handling for various scenarios.
Request
- Method:
POST
- Body: JSON object with key-value pairs representing the input data for the empirical formula calculation.
Response
- Status Codes:
200 OK
: Request was successful, and the empirical formula was calculated.400 Bad Request
: The request was invalid due to one of the following reasons:- The number of elements in the request body exceeds 20.
- The sums of the percentages in the input data do not equal 100.
500 Internal Server Error
: An unknown error occurred while processing the request.
- Response Body:
- On success: A JSON object containing the empirical formula.
- On error: A JSON object containing an error message.
Example Request
{
"H": 20,
"O": 80
}
Example Response
Success Response (200 OK)
{
"ef_formula": "H₄O₁"
}
Error Response (400 Bad Request - Too Many Elements)
{
"error": "The number of elements cannot exceed 20"
}
Error Response (400 Bad Request - Percentages Do Not Sum to 100)
{
"error": "the sums of the percentages is not equal to 100"
}
Error Response (500 Internal Server Error)
{
"error": "Unknown error <error_message>"
}
GET /++calculateCombustionAnalysis
Overview
This service endpoint calculates the empirical formula of a hydrocarbon based on the grams of CO2 and H2O formed from its combustion. It validates the subscription status, handles request parameters, and performs the calculation if the parameters are valid.
Request Parameters
co2
: The grams of carbon dioxide formed as a result of the combustion of the hydrocarbon (required).h2o
: The grams of water formed as a result of the combustion of the hydrocarbon (required).
Response
- Success (200): Returns the empirical formula of the hydrocarbon.
{ "formula": "CH4" }
- Client Error (400): Returns an error message indicating a problem with the request parameters.
{ "error": "Missing co2 grams" }
{ "error": "Missing h2o grams" }
{ "error": "Invalid co2 or h2o parameter values" }
- Unauthorized (401): Returns an error message indicating an invalid subscription.
{ "error": "Subscription invalid" }
- Server Error (500): Returns an error message indicating an unknown error.
{ "error": "Unknown error: detailed error message" }
GET /++chemicalReaction
Description
The ++chemicalReaction
web service processes a chemical formula, checks its validity, and balances the chemical reaction if it is not already balanced.
Request Method
- GET
Endpoint
++chemicalReaction
Parameters
- formula: A string representing the chemical formula to be processed.
Request Headers
- Content-Type:
application/json
Responses
Success Response
- Status Code:
200 OK
- Content-Type:
application/json
- Body: A JSON object containing the original formula, whether it is balanced, the balanced formula (if applicable), reactants, and products.
{
"formula": "H2 + O2 -> H2O",
"is_balanced": false,
"balanced_formula": "2H2 + O2 -> 2H2O",
"reactants": ["2H2", "O2"],
"products": ["2H2O"]
}
Error Responses
Invalid Subscription
- Status Code:
403 Forbidden
- Content-Type:
application/json
- Body: A JSON object containing an error message.
{
"error": "Subscription is not valid"
}
Missing Formula
- Status Code:
400 Bad Request
- Content-Type:
application/json
- Body: A JSON object containing an error message.
{
"error": "Missing formula"
}
Invalid Formula
- Status Code:
400 Bad Request
- Content-Type:
application/json
- Body: A JSON object containing an error message.
{
"error": "Invalid formula"
}
Unknown Error
- Status Code:
500 Internal Server Error
- Content-Type:
application/json
- Body: A JSON object containing an error message and the exception details.
{
"error": "Unknown error {exception details}"
}
POST /++chemicalReaction HTTP/1.1
Host: example.com
Content-Type: application/json
{
"formula": "H2 + O2 -> H2O"
}
HTTP/1.1 200 OK
Content-Type: application/json
{
"formula": "H2 + O2 -> H2O",
"is_balanced": false,
"balanced_formula": "2H2 + O2 -> 2H2O",
"reactants": ["2H2", "O2"],
"products": ["2H2O"]
}
HTTP/1.1 403 Forbidden
Content-Type: application/json
{
"error": "Subscription is not valid"
}
HTTP/1.1 400 Bad Request
Content-Type: application/json
{
"error": "Missing formula"
}
HTTP/1.1 400 Bad Request
Content-Type: application/json
{
"error": "Invalid formula"
}
HTTP/1.1 500 Internal Server Error
Content-Type: application/json
{
"error": "Unknown error {exception details}"
}